Bayesian NetworkΒΆ
Lets start with an example of Bayesian Network. We will create a very small model and perform some simple query.
The network will contain 3 variables connected in a V-shape as shown in the following figure:
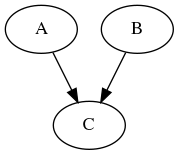
The first thing to do is to declare our Bayesian Network, the variables, and assign the parents for each variable. In this case, all variables are binary.
Note
The BayesianNetwork
is just a wrapper class of the DAGModel<BayesianFactor>
class.
BayesianNetwork model = new BayesianNetwork();
// variables declaration
int A = model.addVariable(2);
int B = model.addVariable(2);
int C = model.addVariable(2);
// parents assignments
model.addParent(C, A);
model.addParent(C, B);
For each variable, the model assign a domain. We need such information to build the factors.
Domain domA = model.getDomain(A);
Domain domB = model.getDomain(B);
Domain domC = model.getDomain(C, A, B);
Finally, there we build a factors for each of the variables. In this example, we show an overview of the many possible ways to instantiate a factor.
BayesianFactor[] factors = new BayesianFactor[3];
factors[A] = new BayesianDefaultFactor(domA, new double[]{.8, .2});
factors[B] = BayesianFactorFactory.factory().domain(domA)
.set(.4, 0)
.set(.6, 1)
.get();
factors[C] = BayesianFactorFactory.factory().domain(domC)
.set(.3, 0, 0, 0)
.set(.7, 0, 0, 1)
.set(.5, 0, 1, 0)
.set(.5, 0, 1, 1)
.set(.4, 1, 0, 0)
.set(.6, 1, 0, 1)
.set(.6, 1, 1, 0)
.set(.4, 1, 1, 1)
.get();
// factor assignment
model.setFactors(factors);
- Factor A
We instantiate a new
BayesianDefaultFactor
from the domain and an array of double values.- Factor B
We use the factory to set the probabilities for states
0
and1
.- Factor C
We use the factory to set the whole joint probability table for variable
C
using the states of all the variables in the domain. In order:C
,A
,B
. Compare the order with the variable order in the definition ofdomC
.
Full example:
package example;
import ch.idsia.crema.core.Domain;
import ch.idsia.crema.factor.bayesian.BayesianDefaultFactor;
import ch.idsia.crema.factor.bayesian.BayesianFactor;
import ch.idsia.crema.factor.bayesian.BayesianFactorFactory;
import ch.idsia.crema.model.graphical.BayesianNetwork;
/**
* Author: Claudio "Dna" Bonesana
* Project: crema
* Date: 27.10.2021 11:33
*/
public class BayesianNetworkExample {
public static void main(String[] args) {
// [1] model declaration
BayesianNetwork model = new BayesianNetwork();
// variables declaration
int A = model.addVariable(2);
int B = model.addVariable(2);
int C = model.addVariable(2);
// parents assignments
model.addParent(C, A);
model.addParent(C, B);
// [2] domains definitions
Domain domA = model.getDomain(A);
Domain domB = model.getDomain(B);
Domain domC = model.getDomain(C, A, B);
// [3] factor definition
BayesianFactor[] factors = new BayesianFactor[3];
factors[A] = new BayesianDefaultFactor(domA, new double[]{.8, .2});
factors[B] = BayesianFactorFactory.factory().domain(domA)
.set(.4, 0)
.set(.6, 1)
.get();
factors[C] = BayesianFactorFactory.factory().domain(domC)
.set(.3, 0, 0, 0)
.set(.7, 0, 0, 1)
.set(.5, 0, 1, 0)
.set(.5, 0, 1, 1)
.set(.4, 1, 0, 0)
.set(.6, 1, 0, 1)
.set(.6, 1, 1, 0)
.set(.4, 1, 1, 1)
.get();
// factor assignment
model.setFactors(factors);
// [4] end
}
}